Jieunny์ ๋ธ๋ก๊ทธ
S2) Unit 2. [JS] ํ๋กํ ํ์ ๋ณธ๋ฌธ
๐ JS๋ ํ๋กํ ํ์ ๊ธฐ๋ฐ์ ์ธ์ด์ด๋ค.
๐ฃ ํ๋กํ ํ์
โ๏ธ ์ํ์ด๋ผ๋ ๋ป (๊ฐ์ฒด์ ์ํ, ์ฆ ๊ฐ์ฒด์ ๋ถ๋ชจ)
โ๏ธ ๊ฐ์ฒด๋ ํ๋กํผํฐ๋ฅผ ๊ฐ์ง ์ ์๋๋ฐ, ํ๋กํ ํ์
์ด๋ผ๋ ํ๋กํผํฐ๋ ๊ฐ์ฒด๊ฐ ์์ฑ๋ ๋ ํ๋กํ ํ์
์ ์ ์ฅ๋ ์์ฑ๋ค์ด ๊ทธ ๊ฐ์ฒด์ ์ฐ๊ฒฐ๋๋ค.
โ๏ธ ๋ชจ๋ ๊ฐ์ฒด๋ ํ๋กํ ํ์
์ ์ ๊ทผํ ์ ์๋ค.
๐ฃ ํ๋กํ ํ์ ๊ณผ ํด๋์ค
class Human {
constructor(name, age) {
this.name = name;
this.age = age;
}
sleep() {
console.log(`${this.name}์ ์ ์ ๋ค์์ต๋๋ค`);
}
}
let kimcoding = new Human('๊น์ฝ๋ฉ', 30);
// ์ค์ตํด๋ณด์ธ์
Human.prototype.constructor === Human; // true
Human.prototype === kimcoding.__proto__; // true
Human.prototype.sleep === kimcoding.sleep; // true
โฐ Human ํด๋์ค์ ์์ฑ์ ํจ์๋ Human์ด๋ค
โฐ Human ํด๋์ค์ ํ๋กํ ํ์
์ Human ํด๋์ค์ ์ธ์คํด์ค์ธ kimcoding์ __proto__ ์ด๋ค.
โ __proto__
์๋ก์ด ๊ฐ์ฒด๋ฅผ ์์ฑํ ๋ ๊ฐ์ฒด์ ํ๋กํผํฐ์ ํจ๊ป __proto__ ํ๋กํผํฐ๊ฐ ๊ฐ์ด ์์ฑ๋๋ค.
__proto__๋ kimcoding์ด๋ผ๋ ๊ฐ์ฒด๋ฅผ ์์ฑํ Human ๊ฐ์ฒด์ prototype ๊ฐ์ฒด๋ฅผ ๊ฐ๋ฆฌํจ๋ค.
โฐ Human ํด๋์ค์ sleep ๋ฉ์๋๋ ํ๋กํ ํ์
์ ์์ผ๋ฉฐ,
Human ํด๋์ค์ ์ธ์คํด์ค์ธ kimcoding์์ kimcoding.sleep์ผ๋ก ์ฌ์ฉํ ์ ์๋ค.
โ๏ธ Human ํด๋์ค์ ์ธ์คํด์ค, ํ๋กํ ํ์
์ ๊ด๊ณ

โ๏ธ Array ํด๋์ค์ ์ธ์คํด์ค, ํ๋กํ ํ์
์ ๊ด๊ณ
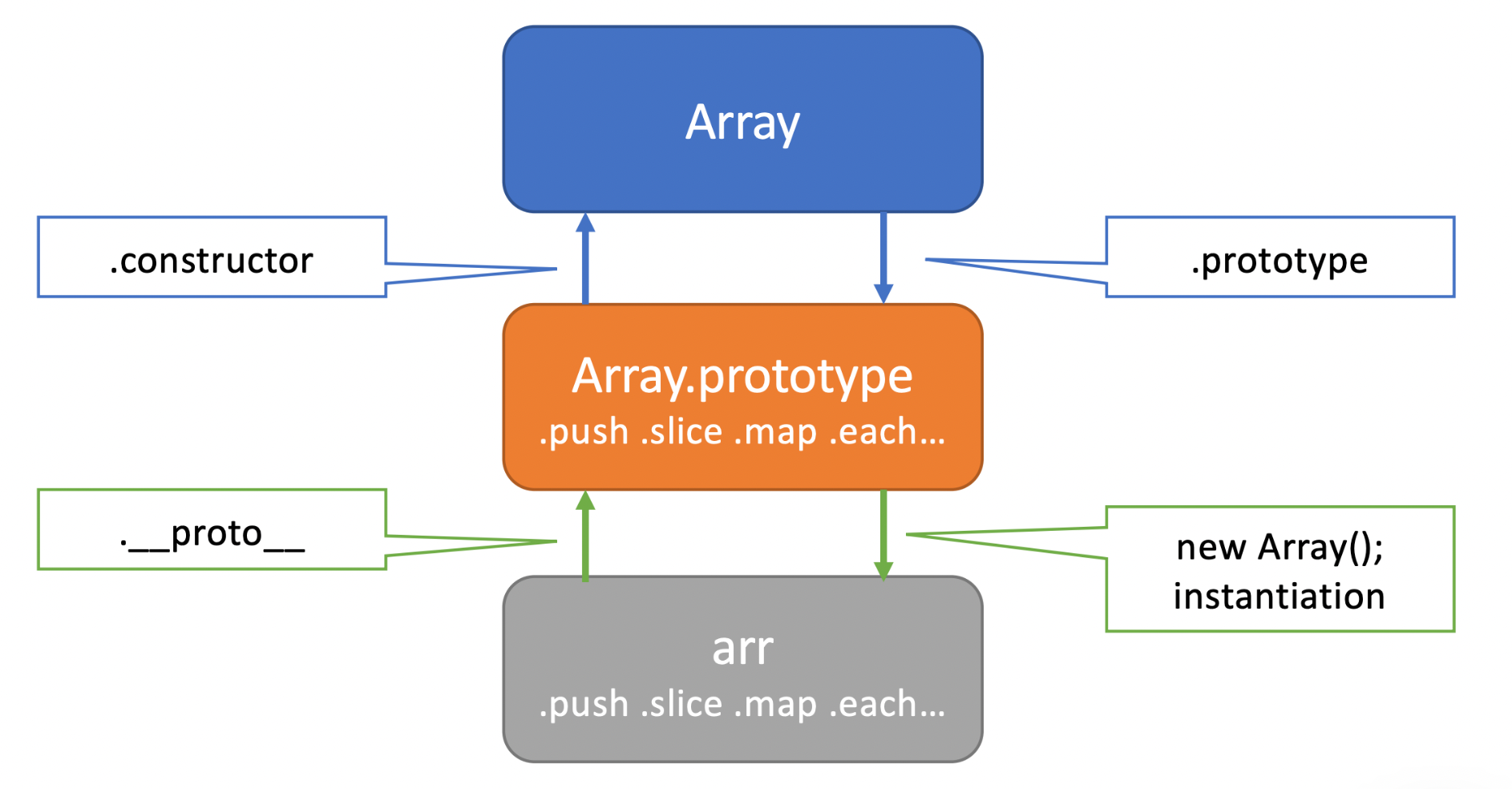
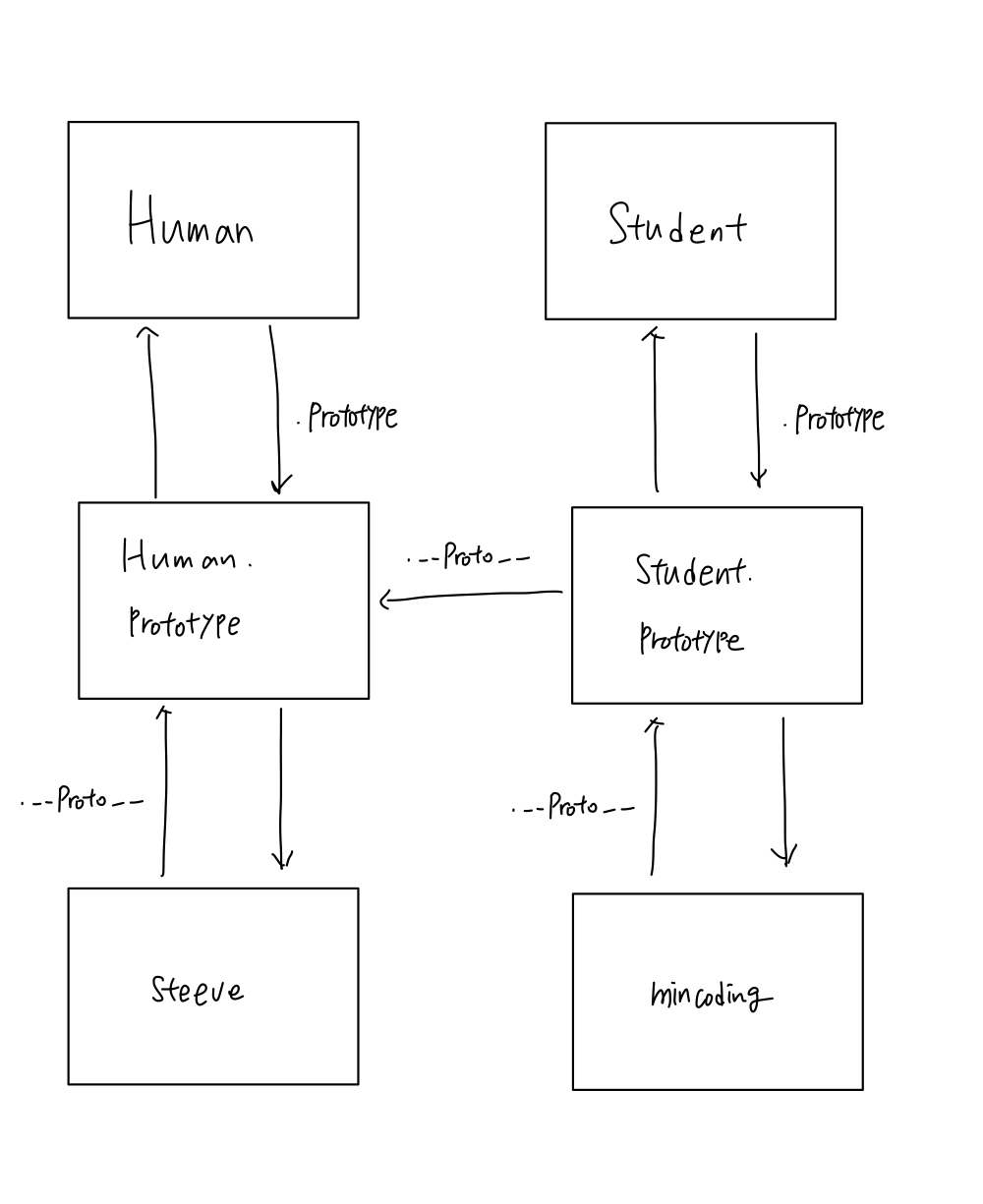
โ๏ธ .prototype์ '์๋'๋ก, .__proto__๋ '์'๋ก ๋ผ๊ณ ์๊ฐํ์.
๐ฃ ํ๋กํ ํ์ ์ฒด์ธ
โ๏ธ JS์์ ์์์ ๊ตฌํํ ๋ ํ๋กํ ํ์ ์ฒด์ธ์ ์ฌ์ฉํ๋ค.
// Human ํด๋์ค
let kimcoding = new Human('๊น์ฝ๋ฉ', 30);
// ์์ฑ
kimcoding.age;
kimcoding.gender;
// ๋ฉ์๋
kimcoding.eat();
kimcoding.sleep();
// Student ํด๋์ค
let parkhacker = new Student('๋ฐํด์ปค', 22);
// ์์ฑ
parkhacker.grade;
// ๋ฉ์๋
parkhacker.learn();
โฐ Student ํด๋์ค๋ Human ํด๋์ค + ์ถ๊ฐ์ ์ธ ํน์ง
โฐ ๋ถ๋ชจ ํด๋์ค(Human) / ์์ ํด๋์ค(Student) => ์ด๋ ๊ฒ ๋ฌผ๋ ค๋ฐ๋ ๊ณผ์ ์ด ์์
โฐ JS์์๋ extends์ super ํค์๋๋ฅผ ํตํด ์์์ ๊ตฌํํ ์ ์๋ค.
// Person ํด๋์ค
class Person {
constructor(first, last, age, gender, interests) {
this.name = {
first,
last
};
this.age = age;
this.gender = gender;
this.interests = interests;
}
greeting() {
console.log(`Hi! I'm ${this.name.first}`);
};
farewell() {
console.log(`${this.name.first} has left the building. Bye for now!`);
};
}
// Teacher ํด๋์ค
class Teacher extends Person {
constructor(first, last, age, gender, interests, subject, grade) {
super(first, last, age, gender, interests);
// subject and grade are specific to Teacher
this.subject = subject;
this.grade = grade;
}
}
โฐ super() : ์์ ํด๋์ค์ ์์ฑ์๋ฅผ ํธ์ถํ์ฌ super()์ ๋งค๊ฐ๋ณ์๋ฅผ ํตํด ์์ ํด๋์ค์ ๋ฉค๋ฒ๋ฅผ ์์๋ฐ์ ์ ์๋ ์ฝ๋
โฐ Teacher์ ์ธ์คํด์ค๋ฅผ ์์ฑํ๋ฉด ์๋ํ๋๋ก Teacher์ Person ์ ์ชฝ์ ๋ฉ์๋์ ์์ฑ์ ์ฌ์ฉํ ์ ์๋ค.
โ๏ธ ๋ฌธ์
let div = document.createElement('div');
div.__proto__.__proto__ // HTMLElement
div.__proto__.__proto__.__proto__// Element
โ๏ธ ๋ฌธ์
class Human {
constructor(name, age) {
this.name = name;
this.age = age;
}
sleep() {
console.log(`${this.name}์ ์ ์ ๋ค์์ต๋๋ค`);
}
}
let kimcoding = new Human('๊น์ฝ๋ฉ', 30);
โฐ Human์ ์ต์์ ํด๋์ค๋ก์จ ๋ถ๋ชจ ํด๋์ค๊ฐ ์๋ค. (โ)
-> ์ต์์ ํด๋์ค๋ Object๋ก, Human์ ๋ถ๋ชจ ํด๋์ค๋ Object ์ด๋ค.
โ๏ธ ๋ฌธ์
let div = document.createElement('div');
document.body.append(div);
div.textContent = '์ฝ๋์คํ
์ด์ธ ์์๋ ๋ชฐ์
์ ์ฆ๊ฑฐ์์ ๋๋ ์ ์์ต๋๋ค.';
div.addEventListener('click', () => console.log('๋ชฐ์
์ ํ๋ฉด ๋๋ผ์ด ์ผ์ด ์๊น๋๋ค.'));
โฐ EventTarget์ prototype์ addEventListener ๋ฉ์๋๊ฐ ์๋ค.
div๋ HTMLDivElement์ ์ธ์คํด์ค์ด๊ณ EventTarget์ ์์๋ฐ์๊ธฐ ๋๋ฌธ์ addEventListener๋ฅผ ์ฌ์ฉํ ์ ์๋ค.
๋ณดํต ํด๋์ค์ ์ธ์คํด์ค๋ new ํค์๋๋ก ์์ฑํ์ง๋ง, DOM์์๋ createElement๋ฅผ ์ฌ์ฉํ๋ค.
โ๏ธ ๋ฌธ์
class Human {
constructor(name, age){
this.name = name;
this.age = age;
}
sleep(){ return `${this.name}์ด(๊ฐ) ์ ์ ์ก๋๋ค.`}
}
class Student extends Human{
constructor(name, age, grade){
super(name, age);
this.grade = grade;
}
study(num){
this.grade = this.grade + num;
return `${this.name}์ ์ฑ์ ์ด ${num}๋งํผ ์ฌ๋ผ ${this.grade}์ด ๋์์ต๋๋ค.`
}
}
class ForeignStudent extends Student{
constructor(name, age, grade, country){
super(name, age, grade);
this.country = country;
}
speak(){
return `${this.country} ์ธ์ด๋ก ์์
์ ์งํํฉ๋๋ค.`
}
}
let americanStudent = new ForeignStudent('jake', 23, 80, '๋ฏธ๊ตญ');
โฐ ForeignStudent์ ๋ถ๋ชจ ํด๋์ค๋ Student, Human ๋ ๊ฐ์ด๋ค.
'CodeStates > learning contents' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
S2) Unit 3. [JS] Node.js (0) | 2023.01.18 |
---|---|
S2) Unit 3. [JS] ๋น๋๊ธฐ(Callback, Promise, Async/Await) (0) | 2023.01.17 |
S2) Unit 2. [JS] ๊ฐ์ฒด ์งํฅ ํ๋ก๊ทธ๋๋ฐ (0) | 2023.01.13 |
S2) Unit 2. [JS] ํด๋์ค์ ์ธ์คํด์ค (0) | 2023.01.13 |
S2) Unit 1. [JS] ๊ณ ์ฐจํจ์ (0) | 2023.01.12 |